MVC模式
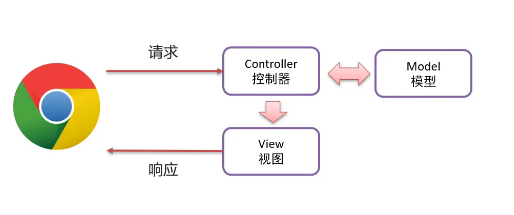
- MVC好处
- 职责单一,互不影响
- 有利于分工协作
- 有利于组件重用
三层架构
- 数据访问层:对数据库的CRUD基本操作(Dao包/Mapper包)
- 业务逻辑层:对业务逻辑进行封装,组合数据访问层中基本功能,形成复杂的业务逻辑功能(Service包)
- 表现层:接收请求,封装数据,调用业务逻辑层,相应数据(web包/controller包)
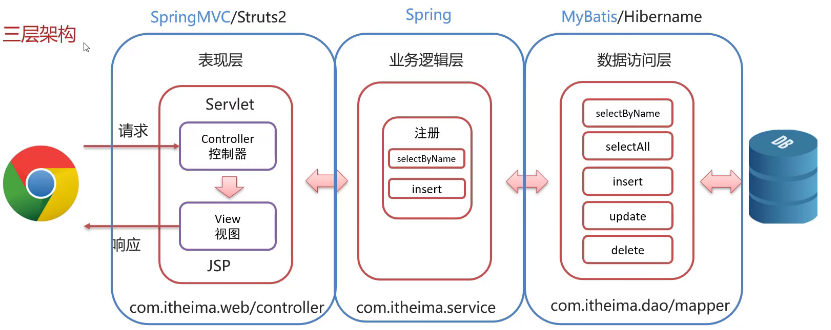
MVC模式和三层架构对照:
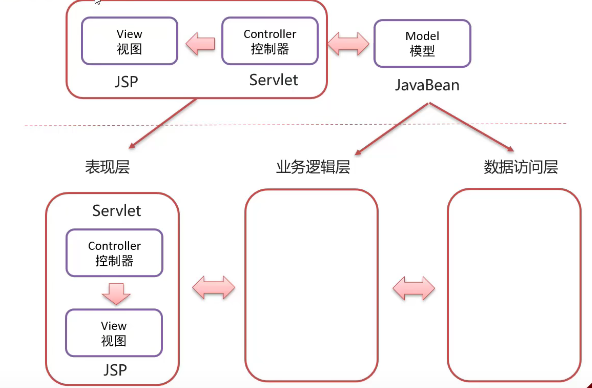
案例:
实现需求:
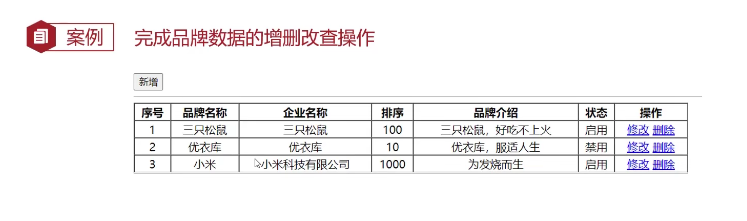
环境准备:
- 创建新的模块brand,引入坐标
- 创建三层架构的包结构
- 数据库表tb_brand
- 实体类Brand
- MyBatis基础环境
- Mybatis-config.xml
- BrandMapper.xml
- BrandMapper接口
过程:
1.首先new一个maven模块brand,配置pom.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75
| <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId> <artifactId>brand</artifactId> <version>1.0-SNAPSHOT</version> <packaging>war</packaging>
<properties> <maven.compiler.source>8</maven.compiler.source> <maven.compiler.target>8</maven.compiler.target> </properties>
<dependencies>
<!--mybatis--> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis</artifactId> <version>3.5.5</version> </dependency>
<!--mysql--> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.34</version> </dependency>
<!--servlet--> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.1.0</version> <scope>provided</scope> </dependency>
<!--jsp--> <dependency> <groupId>javax.servlet.jsp</groupId> <artifactId>jsp-api</artifactId> <version>2.2</version> <scope>provided</scope> </dependency>
<!--jstl--> <dependency> <groupId>jstl</groupId> <artifactId>jstl</artifactId> <version>1.2</version> </dependency> <dependency> <groupId>taglibs</groupId> <artifactId>standard</artifactId> <version>1.1.2</version> </dependency> </dependencies>
<build> <plugins> <plugin> <groupId>org.apache.tomcat.maven</groupId> <artifactId>tomcat7-maven-plugin</artifactId> <version>2.2</version> <configuration> <uriEncoding>UTF-8</uriEncoding> </configuration> </plugin> </plugins> </build>
</project>
|
2.引入webapp和WEB-INF,
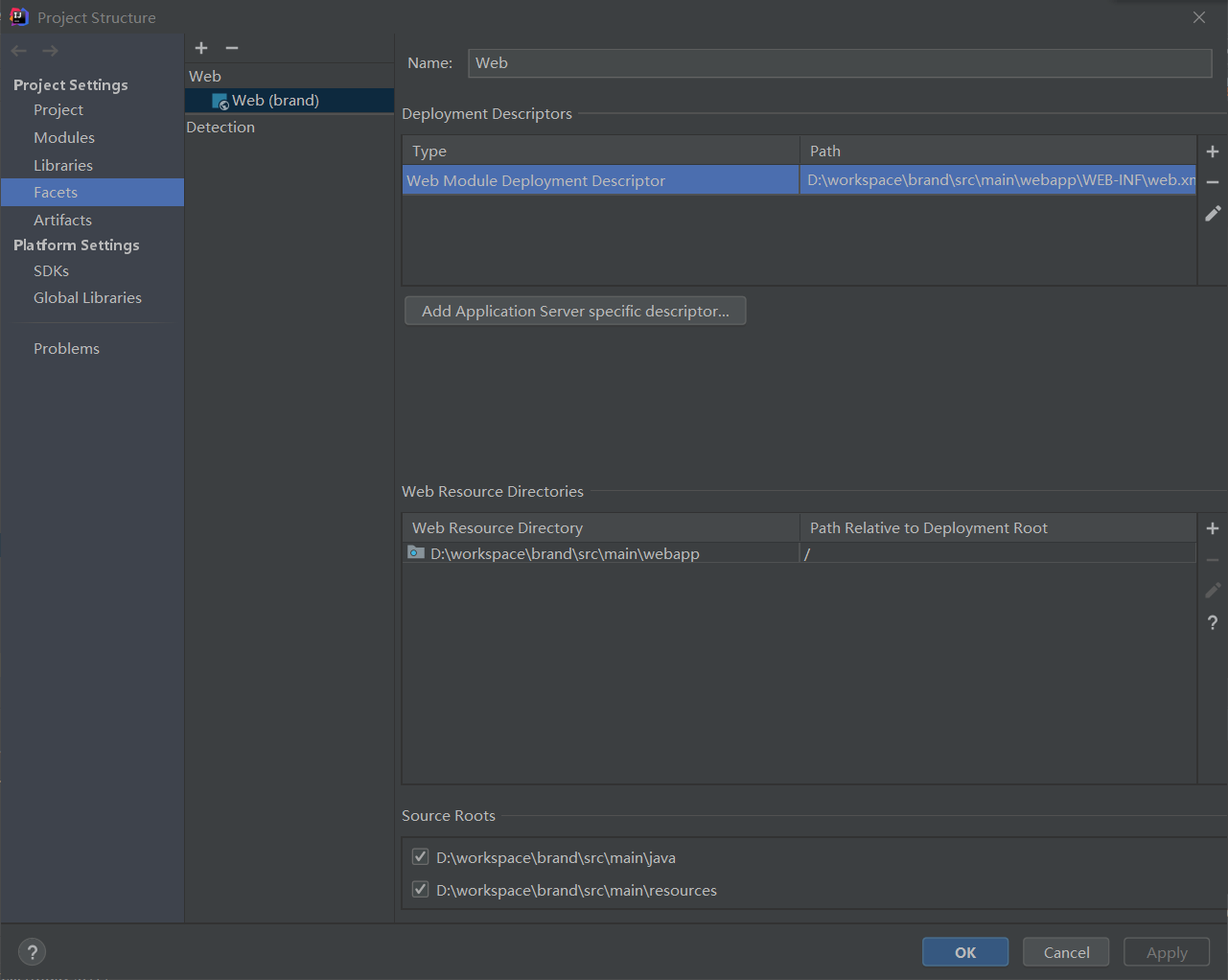
3.创建mysql数据库,创建tb_brand表
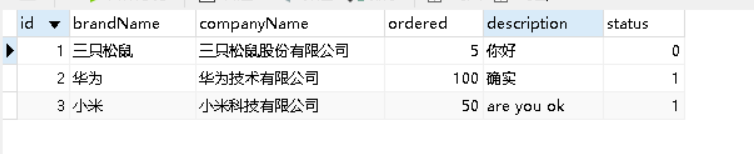
4.创建pojo实体类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100
| package pojo;
public class Brand { private Integer id; private String brandName; private String companyName; private Integer ordered; private String description; private Integer status;
public Brand() { }
public Brand(Integer id, String brandName, String companyName, String description) { this.id = id; this.brandName = brandName; this.companyName = companyName; this.description = description; }
public Brand(Integer id, String brandName, String companyName, Integer ordered, String description, Integer status) { this.id = id; this.brandName = brandName; this.companyName = companyName; this.ordered = ordered; this.description = description; this.status = status; }
public Integer getId() { return id; }
public void setId(Integer id) { this.id = id; }
public String getBrandName() { return brandName; }
public void setBrandName(String brandName) { this.brandName = brandName; }
public String getCompanyName() { return companyName; }
public void setCompanyName(String companyName) { this.companyName = companyName; }
public Integer getOrdered() { return ordered; }
public void setOrdered(Integer ordered) { this.ordered = ordered; }
public String getDescription() { return description; }
public void setDescription(String description) { this.description = description; }
public Integer getStatus() { return status; }
public void setStatus(Integer status) { this.status = status; }
@Override public String toString() { return "Brand{" + "id=" + id + ", brandName='" + brandName + '\'' + ", companyName='" + companyName + '\'' + ", ordered=" + ordered + ", description='" + description + '\'' + ", status=" + status + '}'; } }
|
5.配置dao,service,servlet三层包结构
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| package mapper;
import org.apache.ibatis.annotations.Insert; import org.apache.ibatis.annotations.ResultMap; import org.apache.ibatis.annotations.Select; import pojo.Brand; import java.util.List;
public interface BrandMapper {
@Select("select * from tb_brand") List<Brand> selectAll();
}
|
2.BrandMapper.xml
1 2 3 4 5 6 7
| <?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="mapper.BrandMapper"> </mapper>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| package service;
import mapper.BrandMapper; import org.apache.ibatis.session.SqlSession; import org.apache.ibatis.session.SqlSessionFactory; import pojo.Brand; import util.SqlSessionFactoryUtils;
import java.util.List;
public class BrandService {
SqlSessionFactory factory = SqlSessionFactoryUtils.getSqlSessionFactory();
public List<Brand> selectAll() {
SqlSession sqlSession = factory.openSession();
BrandMapper mapper = sqlSession.getMapper(BrandMapper.class);
List<Brand> brands = mapper.selectAll();
sqlSession.close();
return brands; }
}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| package web;
import pojo.Brand; import service.BrandService;
import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; import java.util.List;
@WebServlet("/selectAllServlet") public class SelectAllServlet extends HttpServlet { private BrandService brandService = new BrandService(); protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
List<Brand> brands = brandService.selectAll();
request.setAttribute("brands",brands);
request.getRequestDispatcher("/brand.jsp").forward(request,response); }
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { this.doGet(request,response); } }
|
util包
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| package util;
import org.apache.ibatis.io.Resources; import org.apache.ibatis.session.SqlSessionFactory; import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import java.io.IOException; import java.io.InputStream;
public class SqlSessionFactoryUtils {
private static SqlSessionFactory sqlSessionFactory;
static {
try { String resource = "mybatis-config.xml"; InputStream inputStream = Resources.getResourceAsStream(resource); sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream); } catch (IOException e) { e.printStackTrace(); } }
public static SqlSessionFactory getSqlSessionFactory(){ return sqlSessionFactory; } }
|
6.Mybatis环境
配置Mybatis,连接本机数据库
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| <?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <!--起别名--> <!--<typeAliases> <package name="pojo"/> </typeAliases>-->
<environments default="development"> <environment id="development"> <transactionManager type="JDBC"/> <dataSource type="POOLED"> <property name="driver" value="com.mysql.jdbc.Driver"/> <property name="url" value="jdbc:mysql:///test?useSSL=false&useServerPrepStmts=true"/> <property name="username" value="root"/> <property name="password" value="xyh"/> </dataSource> </environment> </environments> <mappers> <!--扫描mapper--> <package name="mapper"/> </mappers> </configuration>
|
7.brand.jsp显示页面:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
| <%@ page contentType="text/html;classset = UTF-8" pageEncoding="utf-8" language="java" %> <%@ taglib prefix="c" uri = "http://java.sun.com/jsp/jstl/core" %> <%--<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8" %>--%>
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>SelectAllServlet</title> </head> <body> <input type="button" value="新增" id = "add"> <br> <hr> <table border="1" cellspacing="0" width="80%"> <tr> <th>序号</th> <th>品牌名称</th> <th>企业名称</th> <th>排序</th> <th>品牌介绍</th> <th>状态</th> <th>操作</th>
</tr>
<c:forEach items="${brands}" var = "brand" varStatus="status">
<tr align="center"> <td>${status.count}</td> <td>${brand.brandName}</td> <td>${brand.companyName}</td> <td>${brand.ordered}</td> <td>${brand.description}</td> <c:if test="${brand.status == 1}"> <td>启用</td> </c:if> <c:if test="${brand.status != 1}"> <td>禁用</td> </c:if> <td><a href= "#">修改 </a><a href= "#">删除</a></td> </tr>
</c:forEach>
</table> </hr>
<script> document.getElementById("add").onclick = function () { location.herf = "/brand/addBrand.jsp"; } </script> </body> </html>
|
学习总结:
在三层框架学习中,遇到了很多问题和注意事项,记录一下~
1.路径问题,
Servlet中的@Website注解大小写一定与html需要转发跳转的路径相同。
2.注意打包,因为是web项目,必须打包成war
3.编码问题,启动服务器后发现页面呈现乱码
对于以下这种情况,首先检查浏览器编码是否正确,下载浏览器charset插件,将浏览器页面改为UTF-8编码。
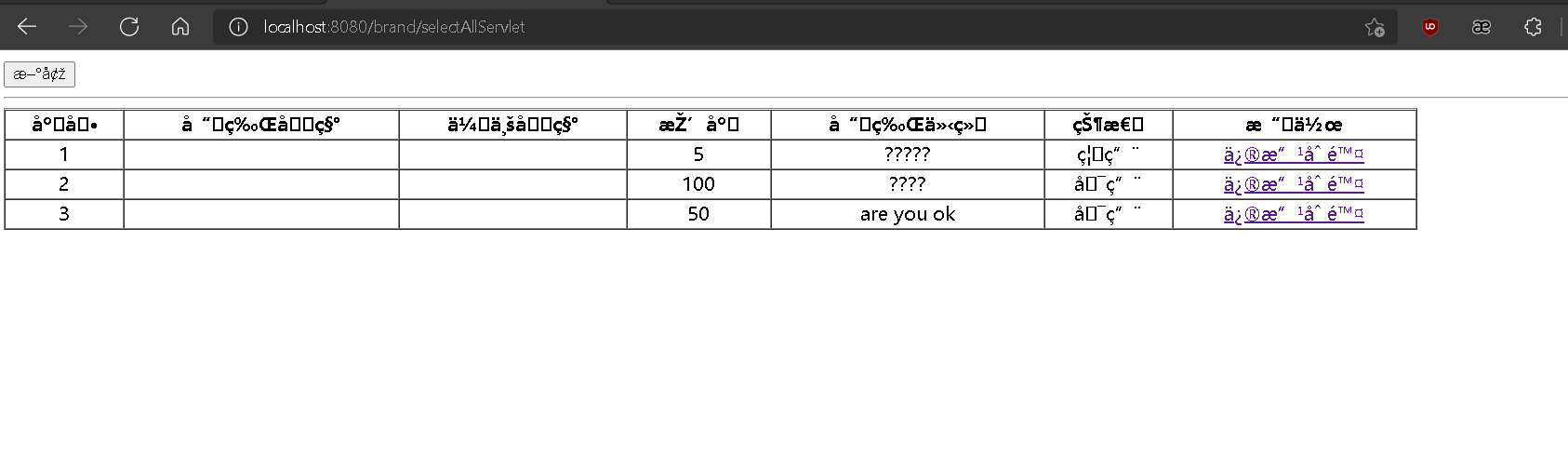
- 修改完浏览器页面编码之后,发现有些地方中文显示问号。

此时,检查自己的数据库编码,tomcat配置文件编码以及IDEA中web项目编码是否均为utf-8,如果都没问题,
大功告成:
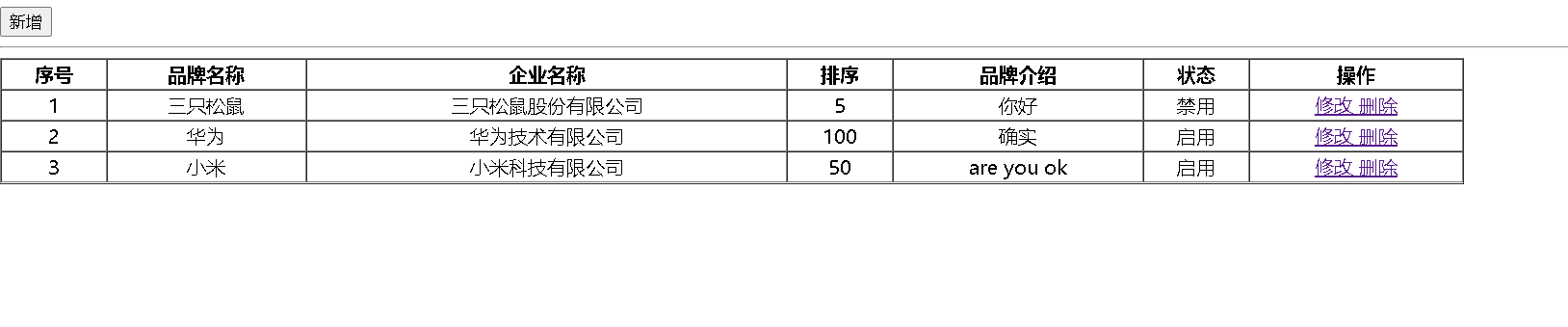
然后,放一下IDEA中的目录结构吧,就当回想以下maven结构了~~
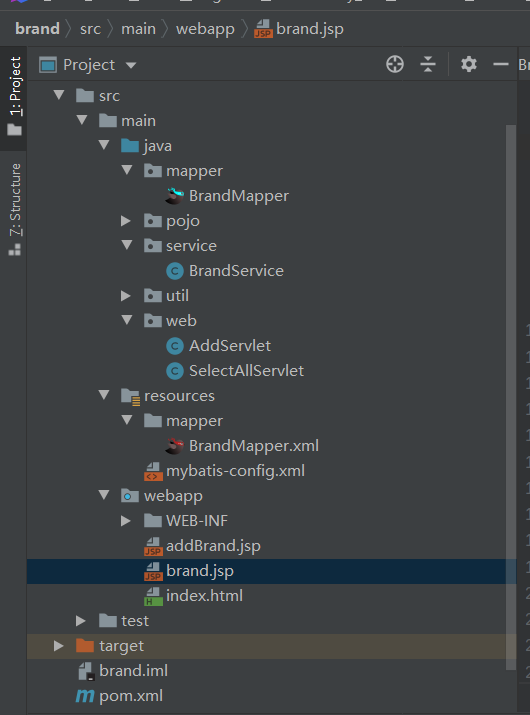
最后,放上帮助我解决编码问题大佬的博客链接:
https://blog.csdn.net/u012611878/article/details/80723491?spm=1001.2101.3001.6650.1&utm_medium=distribute.pc_relevant.none-task-blog-2%7Edefault%7ECTRLIST%7Edefault-1.pc_relevant_paycolumn_v2&depth_1-utm_source=distribute.pc_relevant.none-task-blog-2%7Edefault%7ECTRLIST%7Edefault-1.pc_relevant_paycolumn_v2&utm_relevant_index=2